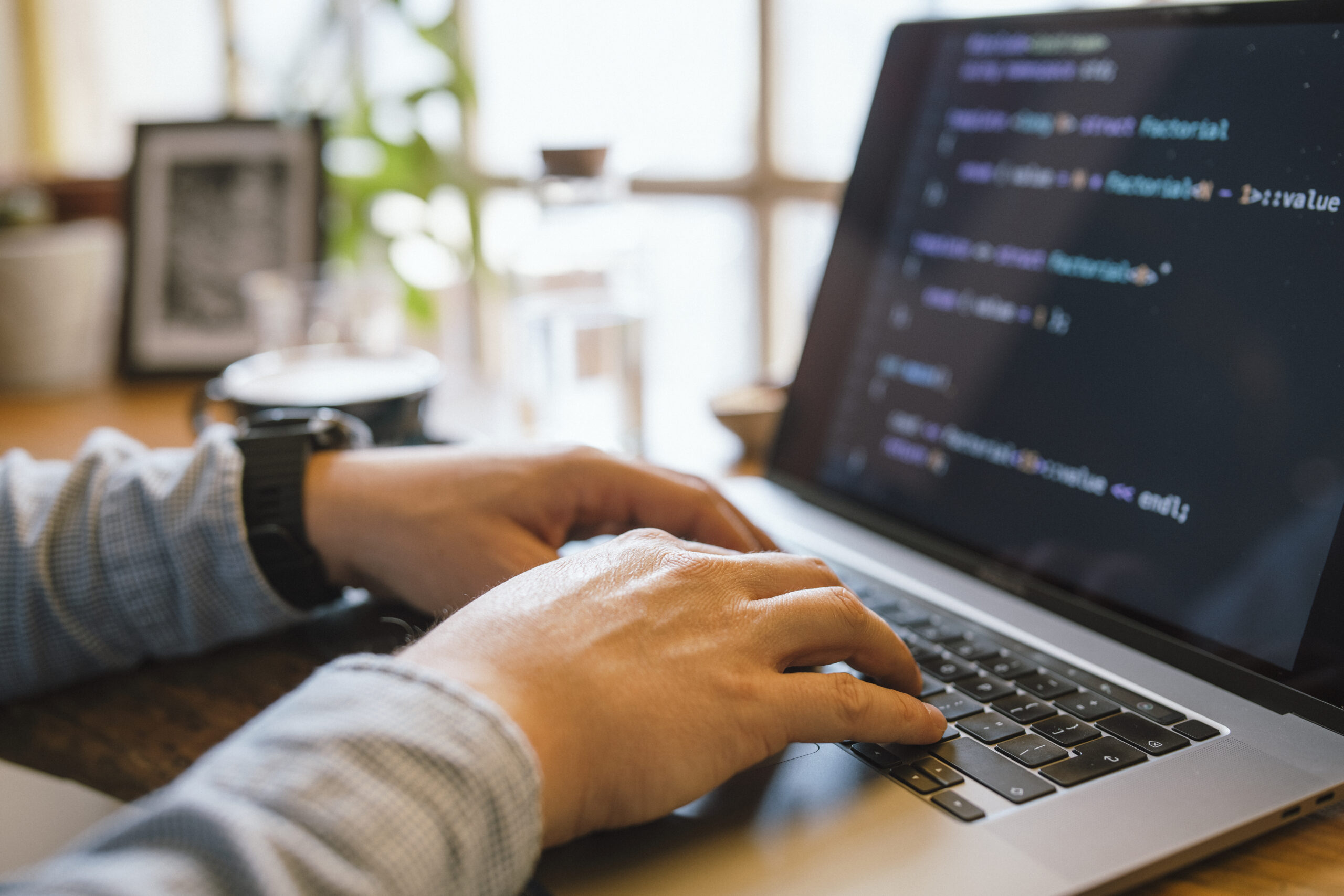
Debugging is Probably the most vital — nonetheless often ignored — techniques in the developer’s toolkit. It isn't really pretty much fixing broken code; it’s about knowledge how and why matters go Mistaken, and Mastering to Assume methodically to unravel complications competently. Whether or not you are a novice or possibly a seasoned developer, sharpening your debugging techniques can help save hrs of stress and dramatically improve your productivity. Here are quite a few procedures that will help developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
On the list of fastest strategies developers can elevate their debugging skills is by mastering the tools they use everyday. Though producing code is one particular part of enhancement, figuring out ways to communicate with it efficiently throughout execution is equally essential. Modern enhancement environments appear equipped with powerful debugging capabilities — but lots of developers only scratch the surface of what these instruments can do.
Take, such as, an Built-in Growth Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code within the fly. When used accurately, they Allow you to notice specifically how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer applications, such as Chrome DevTools, are indispensable for entrance-finish builders. They permit you to inspect the DOM, watch network requests, view true-time functionality metrics, and debug JavaScript in the browser. Mastering the console, resources, and community tabs can flip discouraging UI troubles into workable duties.
For backend or process-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate more than managing procedures and memory management. Finding out these applications could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, grow to be relaxed with version Handle programs like Git to be familiar with code background, locate the precise instant bugs had been launched, and isolate problematic improvements.
Ultimately, mastering your resources signifies likely further than default options and shortcuts — it’s about producing an personal knowledge of your improvement setting making sure that when problems occur, you’re not dropped in the dead of night. The greater you are aware of your applications, the greater time you'll be able to devote fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the situation
Among the most important — and sometimes disregarded — measures in efficient debugging is reproducing the problem. Just before jumping into the code or earning guesses, builders need to have to make a constant surroundings or scenario where the bug reliably seems. With no reproducibility, fixing a bug results in being a video game of prospect, generally resulting in squandered time and fragile code improvements.
The first step in reproducing a problem is collecting just as much context as you possibly can. Talk to inquiries like: What actions triggered The problem? Which atmosphere was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you've, the a lot easier it will become to isolate the precise circumstances less than which the bug happens.
When you’ve gathered sufficient facts, attempt to recreate the trouble in your neighborhood surroundings. This may indicate inputting the same knowledge, simulating similar user interactions, or mimicking program states. If The difficulty appears intermittently, look at writing automated checks that replicate the sting cases or condition transitions associated. These tests not merely help expose the trouble but additionally avert regressions Down the road.
At times, The problem may be surroundings-precise — it'd take place only on selected operating methods, browsers, or beneath individual configurations. Utilizing equipment like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a frame of mind. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're already halfway to repairing it. By using a reproducible circumstance, You may use your debugging applications more successfully, check likely fixes safely and securely, and converse additional Evidently with all your workforce or users. It turns an summary complaint into a concrete challenge — Which’s where by builders prosper.
Read through and Have an understanding of the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Improper. As opposed to seeing them as aggravating interruptions, developers ought to discover to take care of mistake messages as direct communications from the procedure. They frequently tell you what precisely took place, the place it occurred, and sometimes even why it transpired — if you understand how to interpret them.
Start out by looking through the message carefully As well as in total. Numerous builders, particularly when beneath time pressure, look at the initial line and immediately start out producing assumptions. But deeper while in the mistake stack or logs might lie the genuine root result in. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them initially.
Break the mistake down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it stage to a selected file and line quantity? What module or purpose triggered it? These issues can manual your investigation and place you toward the dependable code.
It’s also beneficial to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to acknowledge these can drastically accelerate your debugging course of action.
Some errors are obscure or generic, As well as in Those people instances, it’s very important to examine the context through which the mistake occurred. Examine linked log entries, enter values, and up to date modifications during the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized problems and provide hints about likely bugs.
In the long run, error messages usually are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra effective and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it provides true-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging tactic commences with figuring out what to log and at what stage. Widespread logging stages contain DEBUG, Information, WARN, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard activities (like productive start off-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on key gatherings, condition changes, enter/output values, and demanding conclusion factors inside your code.
Structure your log messages Plainly and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in generation environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, website or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about stability and clarity. That has a well-imagined-out logging solution, you are able to decrease the time it's going to take to spot troubles, attain deeper visibility into your apps, and Increase the General maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it is a sort of investigation. To effectively determine and correct bugs, builders will have to approach the process just like a detective fixing a secret. This state of mind aids break down intricate difficulties into workable pieces and follow clues logically to uncover the root result in.
Start out by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or overall performance difficulties. The same as a detective surveys a criminal offense scene, acquire as much appropriate facts as you could without the need of leaping to conclusions. Use logs, exam conditions, and person stories to piece with each other a clear picture of what’s happening.
Next, variety hypotheses. Ask yourself: What could be causing this behavior? Have any improvements not long ago been manufactured on the codebase? Has this situation occurred prior to under similar instances? The target should be to slender down opportunities and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge within a managed natural environment. In case you suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Enable the outcomes guide you nearer to the truth.
Pay back near attention to smaller specifics. Bugs often cover within the the very least anticipated places—just like a missing semicolon, an off-by-just one error, or maybe a race situation. Be complete and affected person, resisting the urge to patch The difficulty with out thoroughly knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical capabilities, solution issues methodically, and turn into more practical at uncovering hidden problems in intricate units.
Create Exams
Composing checks is one of the best solutions to help your debugging skills and All round growth effectiveness. Assessments don't just help catch bugs early but additionally function a security Internet that provides you assurance when earning changes for your codebase. A effectively-tested application is easier to debug since it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device assessments, which target particular person features or modules. These modest, isolated assessments can promptly expose irrespective of whether a selected bit of logic is Doing work as predicted. Each time a check fails, you instantly know exactly where to look, significantly decreasing the time used debugging. Device tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, integrate integration assessments and stop-to-finish checks into your workflow. These enable make certain that numerous aspects of your software function together efficiently. They’re specifically helpful for catching bugs that happen in elaborate programs with numerous factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Producing tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you need to grasp its inputs, expected outputs, and edge scenarios. This level of knowledge Normally leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails continuously, you'll be able to concentrate on repairing the bug and check out your test move when The difficulty is resolved. This strategy makes sure that a similar bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a disheartening guessing sport into a structured and predictable approach—encouraging you catch much more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging a tricky concern, it’s effortless to be immersed in the condition—watching your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping absent. Getting breaks can help you reset your head, cut down aggravation, and often see the issue from a new perspective.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just hrs earlier. Within this state, your Mind will become a lot less successful at dilemma-fixing. A short walk, a espresso split, and even switching to a special process for 10–15 minutes can refresh your aim. Lots of builders report locating the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, Specifically throughout longer debugging periods. Sitting before a display screen, mentally stuck, is don't just unproductive but in addition draining. Stepping away helps you to return with renewed Strength as well as a clearer mindset. You would possibly instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute split. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below limited deadlines, however it essentially leads to speedier and more effective debugging Eventually.
In short, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you come across is much more than simply A short lived setback—It is a chance to improve to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural difficulty, each one can train you a thing valuable in the event you make time to mirror and assess what went Completely wrong.
Start by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with far better procedures like device screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or knowing and enable you to Create more powerful coding behavior shifting forward.
Documenting bugs can also be an excellent routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you figured out. After a while, you’ll start to see patterns—recurring issues or popular faults—you can proactively keep away from.
In crew environments, sharing Everything you've learned from the bug using your friends could be Particularly powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick know-how-sharing session, supporting Other individuals steer clear of the similar concern boosts team effectiveness and cultivates a more powerful Discovering lifestyle.
A lot more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. After all, many of the very best builders aren't those who create great code, but those that repeatedly discover from their faults.
In the end, each bug you correct provides a brand new layer towards your skill set. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is big. It would make you a far more effective, assured, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.